I found this picture of a ball which is pretty nice because it has a black outline around it, the black helps make motion look much smoother, because you don't have to erase the whole image when moving it a small amount.

Remember: If your saving the image from the web, make sure it's saved in 24-bit color bitmap format.
We can then upload it to the TouchShield using the TSImageUploader program, read on for more.
Steps
1. Enable the TouchShield's image downloading interface
2. Open the image in TSImageUploader
3. Send the image
4. Call up the image in your TouchShield app
Step 1:
We enable the image downloading interface by calling this function on the TouchShield,
image_interface_begin();
This makes the device hold the Arduino in reset while it waits for images from TSImageUploader. Images are sent to the TouchShield at over at 1 MegaBits/sec, thanks USB!
I usually call this as the first thing I do in my setup() function.
Step 2:
The TSImageUploader PC app program does a couple of things, mainly it reformats and sends optimized bitmaps for display on the TouchShield.
Did you know... the TouchShield's OLED hardware connections were optimized for displaying 24-bit bitmaps?! Check out liquidware.org for schematics.
Make sure you remember the name of you bitmap so you can call it up later, duh.
TSImageUploader also can do tricky things to your filenames, so try and keep them to exactly 7 characters in length. If you don't just remember,
ball.bmp becomes "ball[space][space][space]"
and
myNiceBall.bmp becomes "myNiceB"
Allowing larger file names might be a feature supported later, but for now it's something you should remember.
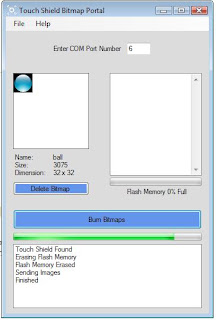
Step 3:
This step is easy, as long as your program is executing the image_interface_begin(); function, then just press the "Burn Bitmaps" button on TSImageUploader. The output window will say when it's finished.
When it's finished uploading, the TouchShield brings the Arduino back out of reset and continues running your app.
Step 4:
Since I named my bitmap's filename ball.bmp, I would call the image up by sending the TouchShield a new app from the Arduino IDE calling this function,
bmp_draw("ball ",10,10);
Which draws ball at location (10, 10) on the screen.
Once your image is downloaded, it is stored in Flash which means it stays there after power cycles.
Remember: Make sure and comment out the image_interface_begin(); function call in subsequent sketches because we don't want to download bitmaps anymore, of course.
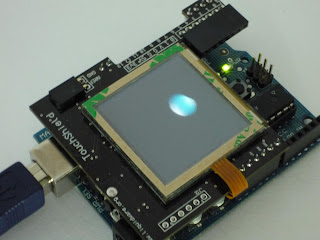
Thats it!
I have attached the code that bounces the ball around the screen below.
Also note that the image is a little messy and leaves a blemish when it's moved to the right. To fix this, you'll see an lcd_rectangle() painting black over a small portion of it's right side removing the blemish left behind after it moves.
Here is the code,
COLOR black = {0,0,0};
char imgSize = 32;
char dirx = 2;
char diry = 1;
char xPos=20;
char yPos=1;
unsigned char xPosPrev = 0;
unsigned char yPosPrev = 0;
void setup()
{
//calling the image downloader
// image_interface_begin();
}
void loop()
{
//move the img
xPos+=dirx;
yPos+=diry;
//off screen check
if ( (xPos > (126-imgSize)) (xPos <>
dirx*=-1;
if ( (yPos > (126-imgSize)) (yPos <>
diry*=-1;
//erase the blemish!
lcd_rectangle((xPosPrev+imgSize),yPosPrev, xPosPrev+imgSize+1,yPosPrev+imgSize, black, black);
//draw the image!
bmp_draw("ball ",xPos,yPos);
//save coordinates
xPosPrev = xPos;
yPosPrev = yPos;
}
3 comments:
In function 'void loop()':
error: expected primary-expression before '>' token
Image uploading does not work. Even initialization fails. I don't know if it's a problem of the usart library.
usart_read_bytes(1); //Wait for us to read a byte
command = buff[0]; //store or read command
this always returns 0x0
I put some lcd_puts to check what is returned...
Are you using Mac or Windows?
If email is easier for you, shoot me a message at avrman@gmail.com
Post a Comment